Routes
Description
- A route defines the different responses that can be sent for an specific request (url and method).
- Routes can contain many variants, which usually define a different response to be sent when the route is requested. The user can choose which variant has to be used by each route on each particular moment.
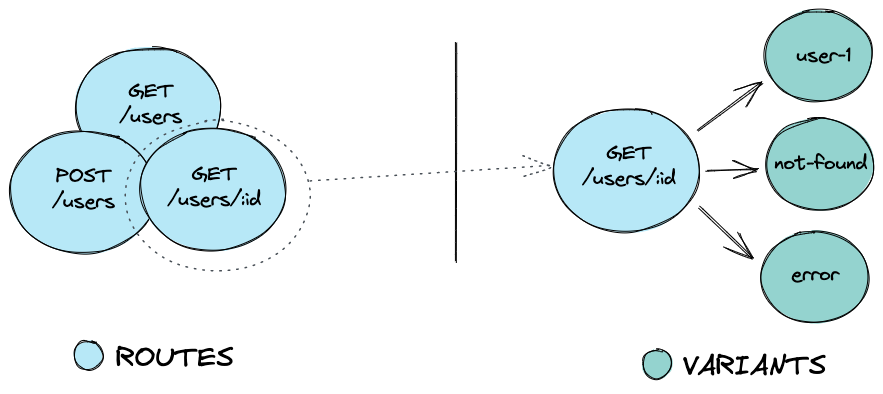
Load
- Usually, routes must be defined in the
mocks/routes
folder of your project. You can organize files inside that folder at your convenience, even creating subfolders, the only rule is that every file must export an array of routes (or a function returning an array of routes). - Files in the
mocks/routes
folder can be of type.json
,.js
,.cjs
,.yml
or even.ts
. Read "Organizing files" and the "Using Babel" guide for further info. - Routes can also be loaded programmatically using the JavaScript API.
- Plugins can provide ways of creating routes automatically. For example, the
openapi
plugin creates routes from OpenAPI documents.
- JS files
- JSON files
- YAML files
- TypeScript files
- JavaScript API
project-root/
├── mocks/
│ ├── routes/ <- DEFINE YOUR ROUTES HERE
│ │ ├── common.js
│ │ ├── books.js
│ │ └── users.js
│ └── collections.js
└── mocks.config.js
project-root/
├── mocks/
│ ├── routes/ <- DEFINE YOUR ROUTES HERE
│ │ ├── common.json
│ │ ├── books.json
│ │ └── users.json
│ └── collections.json
└── mocks.config.js
project-root/
├── mocks/
│ ├── routes/ <- DEFINE YOUR ROUTES HERE
│ │ ├── common.yml
│ │ ├── books.yml
│ │ └── users.yml
│ └── collections.yaml
└── mocks.config.js
project-root/
├── mocks/
│ ├── routes/ <- DEFINE YOUR ROUTES HERE
│ │ ├── common.ts
│ │ ├── books.ts
│ │ └── users.ts
│ └── collections.ts
└── mocks.config.js
Read the using Babel guide for further info about how to use TypeScript.
const { createServer } = require("@mocks-server/main");
const { routes, collections } = require("./fixtures");
const core = createServer();
core.start().then(() => {
const { loadRoutes, loadCollections } = core.mock.createLoaders();
loadRoutes(routes);
loadCollections(collections);
});
Check out the Openapi integration chapter to learn how to create routes automatically from OpenAPI documents 🎉
Format
Routes must be defined as objects containing:
id
(String): Used as a reference for grouping routes into different collections, etc.url
(String|Regexp): Path of the route. Read Routing for further info.method
(String|Array): Method of the request. Defines the HTTP method to which the route will response. It can be also defined as an array of methods, then the route will response to all of them. Valid values are next HTTP methods:GET
,POST
,PUT
,PATCH
,DELETE
,HEAD
,TRACE
andOPTIONS
. Usage of the wildcard*
is also allowed. Read method and multiple methods for further info.delay
(Number): Milliseconds of delay for all variants of this route. This option will override the value of themock.routes.delay
global setting. It can be overridden by thedelay
defined in a variant.variants
(Array): Array of variants. Each variant usually defines a different response to be sent when the route is requested.
Depending on the variant type, the format of the variants may differ. In the next examples we'll see ho to define a variant of type json
, which sends a JSON response. For further info about how to define variants read the next chapter.
- Json
- Yaml
- JavaScript
- Async JS
- TypeScript
[
{
"id": "get-users", // id of the route
"url": "/api/users", // url in path-to-regexp format
"method": "GET", // HTTP method
"variants": [
{
"id": "success", // id of the variant
"type": "json", // variant type
"options": {
"status": 200,
"body": [
{
"id": 1,
"name": "John Doe"
},
{
"id": 2,
"name": "Jane Doe"
}
]
}
},
]
}
]
- id: "get-users"
url: "/api/users"
method: "GET"
variants:
- id: "success"
type: "json"
options:
status: 200
body:
- id: 1
name: "John Doe"
- id: 2
name: "Jane Doe"
const { allUsers } = require("../fixtures/users");
module.exports = [
{
id: "get-users", // id of the route
url: "/api/users", // url in path-to-regexp format
method: "GET", // HTTP method
variants: [
{
id: "success", // id of the variant
type: "json", // variant type
options: {
status: 200,
body: allUsers
}
},
]
}
];
const { getAllUsers } = require("../fixtures/users");
module.exports = async function() {
const allUsers = await getAllUsers();
return [
{
id: "get-users", // id of the route
url: "/api/users", // url in path-to-regexp format
method: "GET", // HTTP method
variants: [
{
id: "success", // id of the variant
type: "json", // variant type
options: {
status: 200,
body: allUsers
}
},
]
}
];
}
import { allUsers } from "../fixtures/users";
const routes = [
{
id: "get-users", // id of the route
url: "/api/users", // url in path-to-regexp format
method: "GET", // HTTP method
variants: [
{
id: "success", // id of the variant
type: "json", // variant type
options: {
status: 200,
body: allUsers
}
},
]
}
];
export default routes;
Routing
Mocks Server uses express
under the hood to create routes, so you can read its docs or the path-to-regexp documentation for further info about how to use routing.
Some basics:
- A single route can match many API urls using parameters:
/users/:id
- Unnamed parameters can be used also:
/*/users
Read the path-to-regexp documentation for further info about how to use routing.
Method
- Requests with a method different to the one defined in the route won't be handled by it. So, you have to define two different routes for handling two different methods of the same URL.
- Valid values are next HTTP methods:
GET
,POST
,PUT
,PATCH
,DELETE
,HEAD
,TRACE
orOPTIONS
(usage of theOPTIONS
method requires some additional configuration). Methods can be also defined using lower case. - The wildcard
*
can also be used as method. In that case, the route would handle all HTTP methods. Read multiple methods for further info.
const { allUsers } = require("../fixtures/users");
module.exports = [
{
id: "get-users", // id of the route
url: "/api/users", // url in path-to-regexp format
method: "GET", // HTTP method
variants: [
{
id: "success", // id of the variant
type: "json", // variant type
options: {
status: 200,
body: allUsers
}
},
]
},
{
id: "create-user", // id of the route
url: "/api/users", // url in path-to-regexp format
method: "POST", // HTTP method
variants: [
{
id: "success", // id of the variant
type: "status", // variant type
options: {
status: 201,
}
},
]
}
];
Note that some types of variants are internally implemented using an Express router instead of an Express middleware. In that case, selecting that variant would produce to ignore the method defined in the route, because, basically, that produces the variant to handle all route methods and subpaths. That is the case of the static
variant handler, for example.
The GET
method will be also called when the request is made with the HEAD
one if no specific HEAD
route is added before for that path.
Multiple methods
The route method can be also defined as an array of methods, then the route will handle requests with any of them.
const { allUsers } = require("../fixtures/users");
module.exports = [
{
id: "modify-user", // id of the route
url: "/api/users/:id", // url in path-to-regexp format
method: ["PATCH", "PUT"] // HTTP methods
variants: [
{
id: "success", // id of the variant
type: "status", // variant type
options: {
status: 200,
}
},
]
},
];
If the route method is not defined, or the wildcard *
is used, then the route will handle requests to any HTTP method:
- Wildcard
- No method
module.exports = [
{
id: "users-error",
url: "/api/users",
method: "*" // All HTTP methods
variants: [
{
id: "error",
type: "status",
options: {
status: 500,
}
},
]
},
];
module.exports = [
{
id: "users-error",
url: "/api/users",
// All HTTP methods, because method is not defined
variants: [
{
id: "error",
type: "status",
options: {
status: 500,
}
},
]
},
];